Canny Edge Detection
The first stage for me is going to edge detection. There are many edge detectors, but I’m going to use the Canny Edge Detector, because I’m vaguely familiar with it and it’s quite well regarded.
Here’s the image I’m going to use for the initial development:
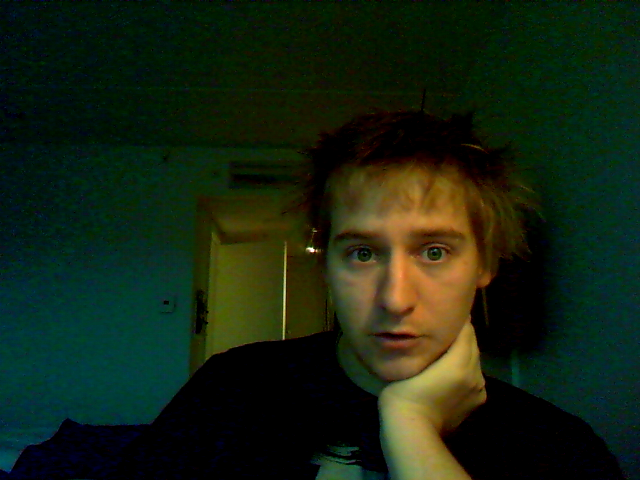
This handsome face *cough* is about to be melted down and turned into a bunch of squiggly lines. It was taken with my webcam in a partially darkened hotel room. On a Sunday. I also look a little shocked for some reason.
Step 1: Noise Reduction
To start with I’m going to blur my image. This might seem a bit counter intuitive, but it’s actually very helpful in cutting down the amount of noise in the picture. I mean, look at it. There’s randomly coloured pixels all over the place, due to poor lighting and a poor webcam. This kind of thing is going to cause interference in the various algorithms I’ll be using. I’ll show off why in a bit.
I’m going to use a very basic “box blur” kernel, not a fancy Gaussian one. Mainly because it’s easier and I’m lazy.
I’ll be using a 3×3 convolution kernel, like this:
This gets applied to an image by moving the centre of the kernel along each pixel in the image, and multiplying the kernel with the window in the image. The result is then summed.
Obviously, you have to deal with edge cases. I’ve taken the easy way out and _not_ dealt with them. I’m only blurring the pixels that are not at the very edge, which leaves a strip around the image that is unprocessed, one pixel thick. Visually, this doesn’t matter- I can leave it there or reduce the image size by 2 pixels in the x and y direction.
Step 2: Get Gradient Intensities:
I’m going to use a basic Sobel filter to perform edge detection. Sobel detects rapid intensity changes in a specific direction. In fact, you need a Sobel filter per direction:
This filter is applied in the same way as the box-blur filter described earlier. Here’s the output:
These two results images are actually from before adding the box-blur filter. I’m doing this whole writeup in the wrong order.
The two gradient intensity images are summed together to get the final gradient result, using this very simple formula
|G| = |Gx| + |Gy|
That is a shortcut from doing the full equation of:
G = SquareRoot(Gx2 + Gy2)
This equation, applied to every pixel in both images, gives this result:
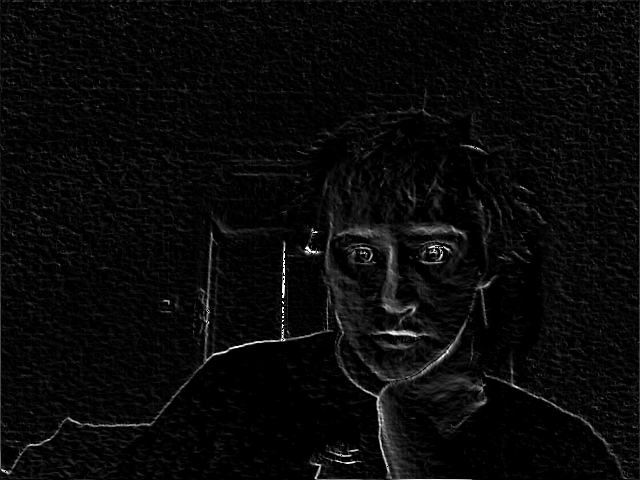
A basic gradient intensity image. By itself, this doesn’t do much, but I like to get tangible output from these algorithms. It’s a good visual lesson for what I just did.
That’s it for now. In the next post I do on this, I’ll be doing:
- Edge direction calculations
- Thin edge detection
- Edge following